Here, a simple calculator design is shown for the demo purpose. This gives understanding of core concept with simple coding. Our final output looks like as shown in the figure. It's design for pc in full screen. Its style may detoriorate in other device however I hope you can fix them later.
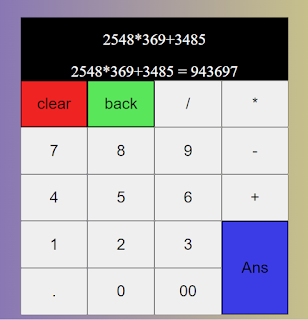 |
Make a Simple Calculator Using HTML, CSS and JavaScript |
Follow the Steps shown:
1. Create a project folder calculator.
2. Open that folder inside your favorite editor. VS code is one I am using.
3. The html, css and javascript code can be placed inside single file: Ex. calculator.html embedding css and javascript code.
4. The file placed inside project folder (must for multiple files for ease) (optional for single file)
5. For easy to display, 3 separate file is created. Name yourself. For convenient the three are Namely, calc.html, calc.css and calc.js
6. Edit calc.html and enter following html.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>
Calculator
</title>
</head>
<body>
<div class="contain">
<!-- <h6>Calculator</h6> -->
<div class="display" id="display" value="0">0</div>
<div class="display" id="display2" value="0">0</div>
<div class="calc_row">
<div><button class="key clear" onclick="cleanSpace()">clear</button></div>
<div><button class="key back" onclick="backSpace()">back</button></div>
<div><button class="key" onclick="myFunction('/')">/</button></div>
<div><button class="key" onclick="myFunction('*')">*</button></div>
</div>
<div class="calc_row">
<div><button class="key" onclick="myFunction('7')">7</button></div>
<div><button class="key" onclick="myFunction('8')">8</button></div>
<div><button class="key" onclick="myFunction('9')">9</button></div>
<div><button class="key" onclick="myFunction('-')">-</button></div>
</div>
<div class="calc_row">
<div><button class="key" onclick="myFunction('4')">4</button></div>
<div><button class="key" onclick="myFunction('5')">5</button></div>
<div><button class="key" onclick="myFunction('6')">6</button></div>
<div><button class="key" onclick="myFunction('+')">+</button></div>
</div>
<div class="calc_row">
<div><button class="key" onclick="myFunction('1')">1</button></div>
<div><button class="key" onclick="myFunction('2')">2</button></div>
<div><button class="key" onclick="myFunction('3')">3</button></div>
<div><button class="key" onclick="evaluate2()"> Ans </button></div>
</div>
<div class="calc_row">
<div><button class="key" onclick="myFunction('.')">.</button></div>
<div><button class="key" onclick="myFunction('0')">0</button></div>
<div><button class="key" onclick="myFunction('00')">00</button></div>
<div><button class="key equal" onclick="evaluate2()"> Ans </button></div>
</div>
</div>
</body>
</html>
7. Edit calc.css and enter following css.
body {
height: 600px;
}
.contain {
margin-top: 3%;
margin-left: 30%;
height: 84%;
margin-right: 0;
width: 40%;
font-size: xx-large;
text-align: center;
}
.calc_row {
display: inline;
width: 100%;
height: 100%;
}
.key {
text-align: center;
width: 25%;
height: 15%;
padding-top: 5%;
padding-bottom: 5%;
float: left;
/* as */
cursor: pointer;
font-size: 2rem;
border: 1px, solid #FFFFFF;
outline: none;
background-color: rbga(255, 255, 255, 0.75);
}
#display,
#display2 {
/* height: 20%; */
padding-top: 5%;
width: 100%;
height: fit-content;
min-height: 12%;
border-color: rgb(0, 0, 0);
background-color: rgb(0, 0, 0);
color: #FFFFFF;
overflow: hidden;
font-family: 'digital-clock-font';
src: url('/public/css/Technology.ttf')
}
.clear {
background-color: rgb(240, 35, 35);
}
.back {
background-color: rgb(90, 230, 90);
}
.equal {
position: absolute;
width: 10%;
height: 29%;
margin-top: 21%;
margin-left: -10%;
background-color: rgb(60, 60, 230);
}
*,
*::before,
*::after {
box-sizing: border-box;
font-family: Gotham Rounded, sans-serif;
font-weight: normal;
}
body {
margin: 0;
padding: 0;
background: linear-gradient(to right, rgb(101, 77, 204), rgb(234, 234, 115));
}
8. Edit calc.js and enter following js code.
document.getElementById("display").innerHTML = "";
document.getElementById("display2").innerHTML = "";
function cleanSpace() {
document.getElementById("display").innerHTML = "";
document.getElementById("display2").innerHTML = "";
}
function backSpace() {
document.getElementById("display").innerHTML = document.getElementById("display").innerHTML.slice(0, -1);
}
function myFunction(string) {
document.getElementById("display").innerHTML = document.getElementById("display").innerHTML + string;
if (!isNaN(document.getElementById("display").innerHTML[document.getElementById("display").innerHTML.length - 1])) {
document.getElementById("display2").innerHTML = eval(document.getElementById("display").innerHTML);
}
}
function evaluate2() {
let finalResult = document.getElementById("display").innerHTML + " = " + document.getElementById("display2").innerHTML;
document.getElementById("display2").innerHTML = finalResult;
}
9. Add link to css file in html.
<link rel="stylesheet" href="calc.css">
10. Add src to js file in html.
<script src=""></script>
11. Placing css content inside html file. In calc html place <style> </style> at the end of head tag. i.e. before </head> and copy and paste your css content inside style tag from calc.css file.(No need if you like to place separately and link). If you already have separate css file and wants to link. Remove link tag and replace by <style> tag.
For separate file for css is used then need to link the css in html using line as shown below.
<link rel="stylesheet" href="calc.css">
or replace by following while using single file to place css content inside html.
<style>
css content
</style>
12. Placing js content inside html file. Place content inside <script> tag. If you already have separate file and link using script src replace that with sript tag and inside place javascript code.
For separate file use below line to link js file in html file.
<script src="calc.js"></script>
or replace by the following while using single file. embedding js code in side script tag.
<script>
js code
</script>
I think the above steps are clear to make simple calculator. If you have any issues further or any suggestion on above please don't forget to write comment below.
Thank you!
Have a good Day!
5 Comments
Elevate your wardrobe game with our timeless leather jackets. fameleatherjackets </a
ReplyDeleteYou carry that jacket with so much confidence—it’s a total game-changer! fameleatherjackets
ReplyDeletebuy this jacket don't miss out it is nearly out of order. Jax teller vest
ReplyDeleteExplore the bold and timeless style of the Women’s Western Jackets collection, where rugged Western charm meets modern elegance. Featuring a range of styles from classic denim to sleek leather, these jackets are perfect for adding a touch of Western flair to your wardrobe. Whether you're dressing up for a night out or keeping it casual, these jackets offer the perfect blend of style, comfort, and durability.
ReplyDeleteFrom luxury waterfront resorts to cozy boutique stays, Vancouver hotels offer something for every traveler. Nestled between mountains and ocean, these accommodations provide stunning views, world-class amenities, and easy access to top attractions. Whether for business or leisure, Vancouver’s hotel scene ensures a comfortable and memorable stay.
ReplyDelete